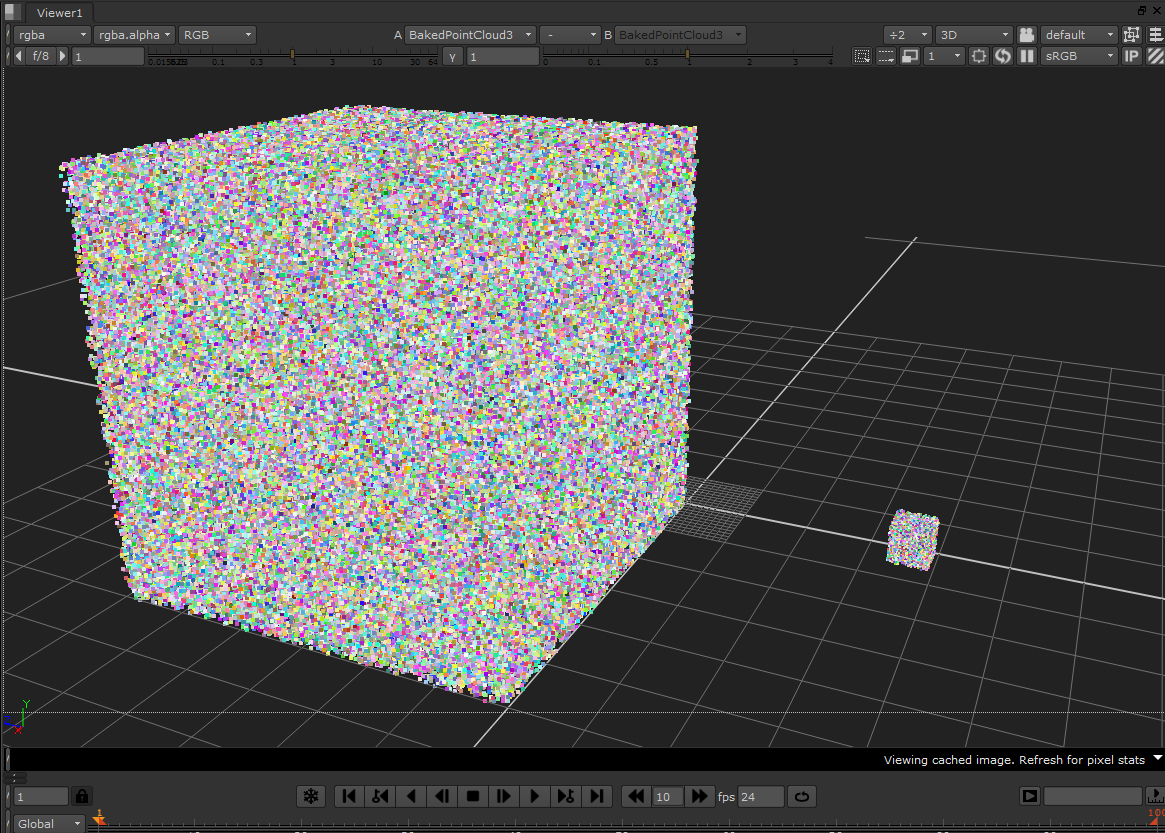
(a 10.000 point cube by a one million point cube)
Generating and managing big 3d data sets inside nuke using python is quite easy using the BakedPointCloud node.
A quick rundown of the node:
set cut_paste_input [stack 0]
version 7.0 v6
BakedPointCloud {
inputs 0
serializeKnob ""
serializePoints "2 1 0 0 2 0 0 "
serializeNormals "2 1 0 0 -1 0 0 "
serializeColors "2 0.0290033 0.0490741 0.100975 0.0290033 0.0490741 0.100975 "
name BakedPointCloud1
label Group1
selected true
xpos 725
ypos 967
}
This is a example of the BakedPointCloud created by the point cloud generator.
We can use this to generate 3D points on the fly (sadly not animated!)
Lets desect it:
set cut_paste_input [stack 0]
version 7.0 v6
BakedPointCloud {
inputs 0
serializeKnob ""
serializePoints "2 1 0 0 2 0 0 " #This part is where the points are stored, first we get the point count, followed by X Y and Z for each point. In this case we have 2 points at 1,0,0 and 2,0,0
serializeNormals "2 1 0 0 -1 0 0 " #This is the normals, and this might not seem interesting at first since its just points, however this can be used for sending off particles into a desired direction.
serializeColors "2 0.0290033 0.0490741 0.100975 0.0290033 0.0490741 0.100975 " #This is the colors, sadly the particle emitter won't sample them
name BakedPointCloud1
label Group1
selected true
xpos 725
ypos 967
}
To sum up, lets say we want to create a single point at position 100,20,-45.2
set cut_paste_input [stack 0]
version 7.0 v6
BakedPointCloud {
inputs 0
serializeKnob ""
serializePoints "1 100 20 45.2 "
serializeNormals "1 1 1 0 "
serializeColors "1 1 0 0"
name BakedPointCloud1
label Group1
selected true
xpos 725
ypos 967
}
I have created this code for generating pointclouds, this example will generate a cube of a million points.
'''================================================================================
; Function: PointClouder(points):
; Description: Generate a pointcloud from a series of specified points
; Parameter(s): points - A list a points formated [[X,Y,Z,VEL_X,VEL_Y,VEL_Z,COL_R,COL_G,COL_B][...]]
; Return: myNode - The pointcloud node created by the function
;
; Note(s): by Mads Hagbarth Lund 2013
;=================================================================================='''
def PointClouder(points):
pc_Points=pc_Velocities=pc_Colors = str(len(points))+ " " #Get the ammount of points
pc_Points = pc_Points + " ".join(str(i) for i in chain1(*points)) #Convert the points from list to clean text
pc_Velocities = pc_Velocities + " ".join(str(i) for i in chain2(*points))
pc_Colors = pc_Colors + " ".join(str(i) for i in chain3(*points))
myNode = nuke.createNode("BakedPointCloud") #Create a empty PointCloud node
myNode.knob("serializePoints").fromScript(pc_Points) #Append the data
myNode.knob("serializeNormals").fromScript(pc_Velocities)
myNode.knob("serializeColors").fromScript(pc_Colors)
return myNode
def chain1(*iterables):
for it in iterables:
for element in it[0:3]:
yield element
def chain2(*iterables):
for it in iterables:
for element in it[3:6]:
yield element
def chain3(*iterables):
for it in iterables:
for element in it[6:9]:
yield element
#Example 2
import random
MyTestPointCloud = []
index = 0
for x in range(0,1000000):
MyTestPointCloud.append([random.uniform(0, 600),random.uniform(0, 600),random.uniform(0, 600),4,5,6,random.uniform(0, 1),random.uniform(0, 1),random.uniform(0, 1)])
PointClouder(MyTestPointCloud)